Sycamore
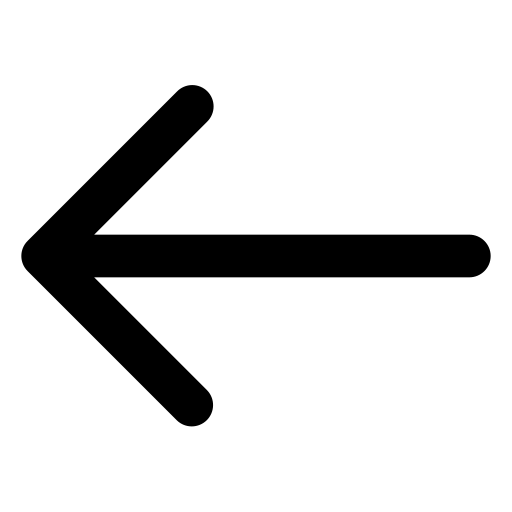
Started | February 2025 |
View on GitHub |
Summary
The culmination of several OpenGL projects and forays into game engine architecture, Sycamore is a 3D game library designed to allow quick, performant, and simple visuals.The goal of the project is to create something which can ship lightweight and interactive 3D experiences to the web, which I may then use for portfolio demonstrations and elements of my personal website. I plan to use Emscripten as a build tool to compile games into web assembly.Example
The video shown above is built from the following C++ code alone:#include <iostream>#include <memory>#include <string>#include "Sycamore.h"class TGame : public scm::TApp{typedef scm::TApp Super;public:TGame(const char* name, unsigned int width, unsigned int height): Super(name, width, height){ }};class TPrototypeScene : public scm::TScene{typedef scm::TScene Super;public:void BeginPlay() override{// Load assetsscm::TAssetManager::Directory = "../assets/";auto material = scm::TAssetManager::Load<scm::TMaterial>("flat.glsl");// Create cubeauto geom (scm::TAssetManager::Load<scm::TGeometry>("cube.obj"));auto mesh = std::make_shared<scm::TMeshComponent>(geom, material);cube = new scm::TMesh(mesh);Super::BeginPlay();}void Render() override{Renderer.Draw(*cube);}scm::TMesh* cube;};int main(){std::cout << "Running game" << std::endl;TGame* game = new TGame("Hello Cube", 1920U, 1080U);game->UseScene<TPrototypeScene>();game->Start();}